sorting problem
-
- Ice Cold
- Posts: 202
- Joined: 2008.09.26 (11:49)
- Location: Australia
- Contact:
so I have a point - say, a camera - and I have many other points (enemies, geometry objects, whatever). imagine there's a ray coming out of the camera which slowly turns, I want to sort the points based on the order the ray would pass them.
I don't know anything about computerized sorting. Something I can initially think of is to convert all the points to angles from the camera, and order them with flash's built in array sorting function, but then I can't think of any good way to transfer this sorted order to the original list of points. Plus, converting points to angles requires trig, and i'd prefer to be able to do this with lots of points so I'd want it reasonably fast.
Any ideas? Am I being clear enough?
- Bayking
- Posts: 315
- Joined: 2008.10.01 (20:26)
- NUMA Profile: http://nmaps.net/user/exuberance
- Location: Guelph, Ontario, Canada
As for getting teh sorted order to the original list of points, you could change your object for points to also include a 3rd variable: the angle it makes with the camera. Then sort that array of objects directly. Here's code for a function I wrote a while ago in Actionscript 2.0 for sorting a list of objects in ascending order based on 1 property of the objects:
NOTE: This is Actionscript 2.0. If you need Actionscript 3.0, you might have to adjust a few things. I know you could change the Number type vars to int to save on memory and :Void becomes :void in AS3. Beyond that, I'm not sure what you need to change. My trial version for the new Flash ran out :(
Code: Select all
/*
* call this function with 2 arguments, array and varname
* Args:
* array:Array an array of objects with a common variable that you wish to search
* varname:String the name of the variable you want the objects to be sorted by. This is a string
* Returns: void
* Side effects: the array has been sorted by the indicated variable
* example: A is an array of objects containing 2 variables _x and _y. To sort the objects in ascending order of _x value use
* quickSort_objectArray(A,"_x");
*/
function quickSort_objectArray(array:Array, varname:String, low:Number, high:Number):Void {
var bottom:Number;
var top:Number;
var pivot;
var temp:Object;
if (arguments.length <= 2) {
low = 0;
high = array.length - 1;
}
if (high > low) {
pivot = array[high][varname];
bottom = low - 1;
top = high;
while (true) {
while ((array[++bottom][varname] < pivot) && (bottom < array.length));
while ((array[--top][varname] > pivot) && (top > 0));
if (bottom >= top) {
break;
}
temp = array[bottom];
array[bottom] = array[top];
array[top] = temp;
}
temp = array[bottom];
array[bottom] = array[high];
array[high] = temp;
quickSort_objectArray(array, varname, low, bottom - 1);
quickSort_objectArray(array, varname, bottom + 1, high);
}
}
Comic Activity-O-Meter: (how often I'm updating my comic)
NOTE: If I just add a bunch of comics in one day, but plan on going back to normal after that, I probably won't update the status.
+ Dead: Canceled. Done. Maybe you'll get a random comic like once a year, but it's pretty much done.
- Zombie (Dead/Comatose): The comic is probably done regular updates forever, but I'll probably still add something once in a blue moon. It's still POSSIBLE, that I'll raise the status up, but not very likely. Maybe I'll have a comicplosion for like a week, then go back to being dead
+ Comatose: Complete stand-by. No (or very few) updates for some amount of time, but the comic's far from being over
- <AFK> (Comatose/Loitering): Stand-by, but you might possibly count on a few updates once and a while. Again, this is temporary
+ Loitering: Like comatose, but for short amount of times.
- Turtling (Loitering/Semi-Active): Really slooooww updates
+ Semi-Active: One every 2 weeks...ish?
- Quasi-Active (Semi-Active/Active): Averaging about 2 comics every 3 weeks
+ Active: Loosely defined status, but about a weekly update
- Over-Active (Active/Power-leveling): About 2 comics a week
+ Power-leveling: About 3 comics a week. Possible a schedule, possibly not
- Über-Epic (Power-leveling/COMICPLOSION!!): In some cases, this may actually be mean updates more frequently than COMICPLOSION!!, but I'm defining this level as a non-organized comic rush, kind of like a few days after my comic started
+ COMICPLOSION!!: Daily updates for a minimum of 5 days (since the daily updates started. It remains at this status until the 5, 7, whatever days are done)

"Science without religion is lame. Religion without science is blind." ~Albert Einstein
My N+ Vector Sprite Sheet ::: My Caption Contest ::: My Comic :::Puzzles of the Exuberant ::: DEFEND YOUR NINJA: THE FLASH GAME (Release Date TBA)

Exüberance on WoW
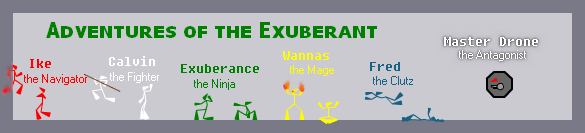
-
- Ice Cold
- Posts: 202
- Joined: 2008.09.26 (11:49)
- Location: Australia
- Contact:
Specifically, I want to do something like http://metanet.2.forumer.com/index.php?showtopic=20674
It's an idea I gave up on before, but now my physics engine is nicer so I can just cast rays to all my corner points in geometry and join them up to represent visually the vision cone. The issue is, I need to order the points before joining them up.
I looked at your code, it's not making any sense to me right now but it works, so I'll try and make sense of it later. Is this considered fast for a sorting algorithm?
Sorting like this is not ideal because I wanted to avoid trig, but I can't see any way that could feasibly not involve trig so i'll use this for now. Thanks heaps!
- Bayking
- Posts: 315
- Joined: 2008.10.01 (20:26)
- NUMA Profile: http://nmaps.net/user/exuberance
- Location: Guelph, Ontario, Canada
Would it be possible to construct your function such that it finds the corner points in order so you don't have to sort it? I don't think it would be, but it's hard to say.
Comic Activity-O-Meter: (how often I'm updating my comic)
NOTE: If I just add a bunch of comics in one day, but plan on going back to normal after that, I probably won't update the status.
+ Dead: Canceled. Done. Maybe you'll get a random comic like once a year, but it's pretty much done.
- Zombie (Dead/Comatose): The comic is probably done regular updates forever, but I'll probably still add something once in a blue moon. It's still POSSIBLE, that I'll raise the status up, but not very likely. Maybe I'll have a comicplosion for like a week, then go back to being dead
+ Comatose: Complete stand-by. No (or very few) updates for some amount of time, but the comic's far from being over
- <AFK> (Comatose/Loitering): Stand-by, but you might possibly count on a few updates once and a while. Again, this is temporary
+ Loitering: Like comatose, but for short amount of times.
- Turtling (Loitering/Semi-Active): Really slooooww updates
+ Semi-Active: One every 2 weeks...ish?
- Quasi-Active (Semi-Active/Active): Averaging about 2 comics every 3 weeks
+ Active: Loosely defined status, but about a weekly update
- Over-Active (Active/Power-leveling): About 2 comics a week
+ Power-leveling: About 3 comics a week. Possible a schedule, possibly not
- Über-Epic (Power-leveling/COMICPLOSION!!): In some cases, this may actually be mean updates more frequently than COMICPLOSION!!, but I'm defining this level as a non-organized comic rush, kind of like a few days after my comic started
+ COMICPLOSION!!: Daily updates for a minimum of 5 days (since the daily updates started. It remains at this status until the 5, 7, whatever days are done)

"Science without religion is lame. Religion without science is blind." ~Albert Einstein
My N+ Vector Sprite Sheet ::: My Caption Contest ::: My Comic :::Puzzles of the Exuberant ::: DEFEND YOUR NINJA: THE FLASH GAME (Release Date TBA)

Exüberance on WoW
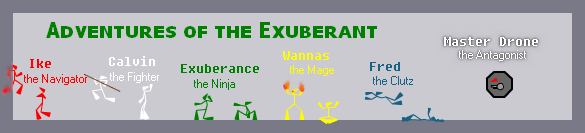
-
- Ice Cold
- Posts: 202
- Joined: 2008.09.26 (11:49)
- Location: Australia
- Contact:
I now understand the quicksort, thanks. I can understand that it's very close to the upper limit on speed for my fairly random data, and it doesn't create enough of an impact on the processing required each step for me to worry about it further.
- Global Mod
- Posts: 1416
- Joined: 2008.09.26 (05:35)
- NUMA Profile: http://nmaps.net/user/scythe33
- MBTI Type: ENTP
- Location: 09 F9 11 02 9D 74 E3 5B D8 41 56 C5 63 56 88 C0
(technically, you're using trig here, but you're not calculating the angle, which saves a good bit of time)
-
- Ice Cold
- Posts: 202
- Joined: 2008.09.26 (11:49)
- Location: Australia
- Contact:
I thought I'd need to mess with divide-by-zero stuff, but flash conveniently has an "Infinity" value which is a pain everywhere else but actually useful here.
Who is online
Users browsing this forum: Google [Bot] and 2 guests